Waifu2x
Waifu2x is an algorithm that upscales images while reducing noise within the image. It gets its name from the anime-style art known as 'waifu' that it was largely trained on. Even though waifus made up most of the training data, this waifu2x api still performs well on photographs and other types of imagery. You can use Waifu2x to double the size of your images while reducing noise.
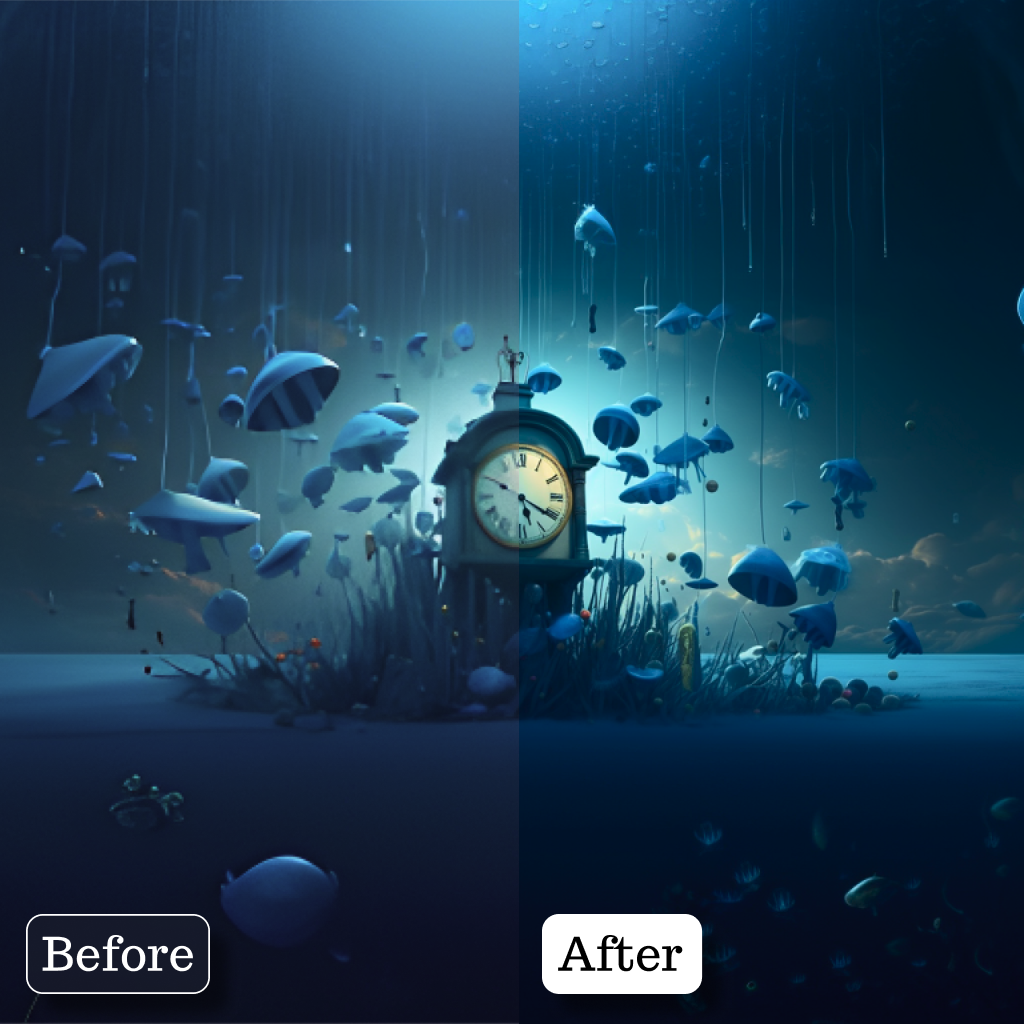